I’ve been working on a flexible table API generator for Oracle Databases for several months. A TAPI generator doesn’t sound like a real innovation. But this one contains some features you probably have not seen before in the TAPI generator and hopefully will like it as much as I do.
In this post, I will not explain the feature set thoroughly. Instead, I will more or less focus on one of my favourite features.
Four models
The generator knows the following four data models.
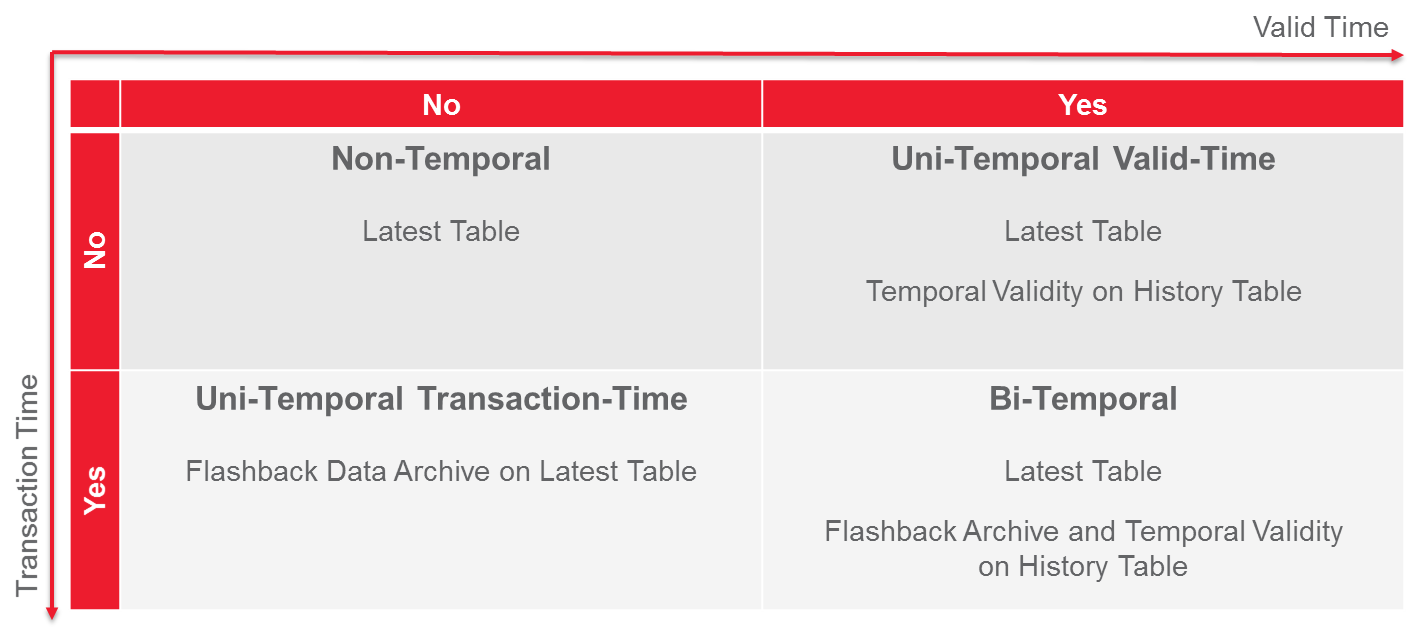
If your table is based on one of these four models you may
- simply generate a table API for it or
- switch to another model and optionally generate a table API as well.
Option 2) is extraordinary, since it will preserve the existing data. E.g. it will preserve the content of the flashback data archive when you switch your model from uni-temporal transaction time to a bi-temporal model even if the flashback archive tables need to be moved to another table. Furthermore, it will keep the interface for the latest table the same. No application change is required. Everything with just a few mouse clicks. If this sounds interesting to you, then have a look at https://github.com/oddgen/bitemp/blob/main/README.md where the concept is briefly explained or join me in my session “oddgen – Bi-temporal Table API in Action” at the More than just – Performance Days 2016. Remote participation is still possible.
Option 1) is what we had for years. It was part of Oracle Designer, it’s part of SQL Developer in a simplified way and there are some more or less simple table API generators around. So no big deal. However, when you choose option 1), there is one cool part. The hook API package concept.
The Hook API
The problem with a lot of table API solutions is, that there is typically no developer-friendly way to include the business logic. I’ve seen the following:
- Manual changes of the generated code, which is for various reasons not a good solution.
- External hooks, e.g. in XML files, INI files, relational tables, etc. and merged at generation time into the final code. Oracle Designer worked that way.
- Code which is dynamically executed by the generator at runtime, e.g. code snippets is stored in a pre-defined way in relational tables.
But what I’ve never seen, was business logic implemented in manually crafted PL/SQL packages, separated from the PL/SQL generated code. That’s strange because this is a common practice in Java-based projects.
In Java, you typically define an interface for that and configure at runtime the right implementation. In PL/SQL we may do that similarly. A PL/SQL specification is an interface definition. That just one implementation may exist for an interface is not a limiting factor in this case.
Bitemp Remodeler generates the following hook API package specification for the famous EMP table in schema SCOTT:
CREATE OR REPLACE PACKAGE emp_hook AS
/**
* Hooks called by non-temporal API for table emp_lt (see package body of emp_api)
* generated by Bitemp Remodeler for SQL Developer.
* The body of this package is not generated. It has to be crafted and maintained manually.
* Since the API for table emp_lt ignores errors caused by a missing hook package body, the implementation is optional.
*
* @headcom
*/
/**
* Hook called before insert into non-temporal table emp_lt.
*
* @param io_new_row new Row to be inserted
*/
PROCEDURE pre_ins (
io_new_row IN OUT emp_ot
);
/**
* Hook called after insert into non-temporal table emp_lt.
*
* @param in_new_row new Row to be inserted
*/
PROCEDURE post_ins (
in_new_row IN emp_ot
);
/**
* Hook called before update non-temporal table emp_lt.
*
* @param io_new_row Row with updated column values
* @param in_old_row Row with original column values
*/
PROCEDURE pre_upd (
io_new_row IN OUT emp_ot,
in_old_row IN emp_ot
);
/**
* Hook called after update non-temporal table emp_lt.
*
* @param in_new_row Row with updated column values
* @param in_old_row Row with original column values
*/
PROCEDURE post_upd (
in_new_row IN emp_ot,
in_old_row IN emp_ot
);
/**
* Hook called before delete from non-temporal table emp_lt.
*
* @param in_old_row Row with original column values
*/
PROCEDURE pre_del (
in_old_row IN emp_ot
);
/**
* Hook called after delete from non-temporal table emp_lt.
*
* @param in_old_row Row with original column values
*/
PROCEDURE post_del (
in_old_row IN emp_ot
);
END emp_hook;
/
The generated table API calls before an INSERT the pre_ins procedure and after the INSERT the post_ins procedures. For DELETE and UPDATE this works the same way. On the highlighted line 5 and 6 two interested things are pointed out. The body is not generated and the body does not need to be implemented since the API ignores errors caused by a missing PL/SQL hook package body.
Technically this is solved as follows in the API package body:
e_hook_body_missing EXCEPTION;
PRAGMA exception_init(e_hook_body_missing, -6508);
<<pre_ins>>
BEGIN
emp_hook.pre_ins(io_new_row => l_new_row);
EXCEPTION
WHEN e_hook_body_missing THEN
NULL;
END pre_ins;
do_ins(io_row => l_new_row);
Now you may ask what the performance impact of these e_hook_body_missing exceptions are. I’ve done a small test and called a procedure without and with implemented body 1 million times. The overhead of the missing body exception is about 7 microseconds per call. Here’s the test output from SQL Developer, the relevant lines 51 and 89 are highlighted.
SQL> SET FEEDBACK ON
SQL> SET ECHO ON
SQL> SET TIMING ON
SQL> DROP PACKAGE dummy_api;
Package DUMMY_API dropped.
Elapsed: 00:00:00.027
SQL> DROP PACKAGE dummy_hook;
Package DUMMY_HOOK dropped.
Elapsed: 00:00:00.030
SQL> CREATE OR REPLACE PACKAGE dummy_hook AS
PROCEDURE pre_ins;
END dummy_hook;
/
Package DUMMY_HOOK compiled
Elapsed: 00:00:00.023
SQL> CREATE OR REPLACE PACKAGE dummy_api AS
PROCEDURE ins;
END dummy_api;
/
Package DUMMY_API compiled
Elapsed: 00:00:00.034
SQL> CREATE OR REPLACE PACKAGE BODY dummy_api AS
e_hook_body_missing EXCEPTION;
PRAGMA exception_init(e_hook_body_missing, -6508);
PROCEDURE ins IS
BEGIN
BEGIN
dummy_hook.pre_ins;
EXCEPTION
WHEN e_hook_body_missing THEN
NULL;
END pre_ins;
dbms_output.put('.');
END ins;
END dummy_api;
/
Package body DUMMY_API compiled
Elapsed: 00:00:00.040
SQL> -- without hook body
SQL> BEGIN
FOR i IN 1..1E6 LOOP
dummy_api.ins;
END LOOP;
END;
/
PL/SQL procedure successfully completed.
Elapsed: 00:00:07.878
SQL> CREATE OR REPLACE PACKAGE BODY dummy_hook AS
PROCEDURE pre_ins IS
BEGIN
dbms_output.put('-');
END pre_ins;
END dummy_hook;
/
Package body DUMMY_HOOK compiled
Elapsed: 00:00:00.029
SQL> -- with hook body
SQL> BEGIN
FOR i IN 1..1E6 LOOP
dummy_api.ins;
END LOOP;
END;
/
PL/SQL procedure successfully completed.
Elapsed: 00:00:00.632
It makes sense to provide a body with a NULL implementation to avoid the small overhead of handling the missing body exception.
Nonetheless, the way the business logic is separated from the generated code is one of the many things I like about Bitemp Remodeler.
Download Bitemp Remodeler from the Download section on my blog or install it directly via the SQL Developer update site http://update.oddgen.org/